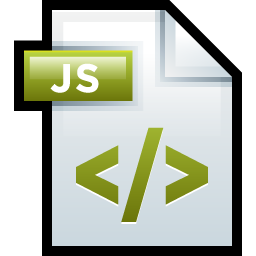
HTML5 Browser Compatibility
HTML5 is the future of web development, because of its capabilities for providing media rich content on multiple web and mobile platforms.
At the core of this new technology is JavaScript, for which there are numerous frameworks for assembling enterprise applications.
Due to the fact that JavaScript, HTML, and CSS interpretation are the responsibility of the browser, this has lead and continues to lead to compatibility issues with
web systems between different browsers.
What techniques are most effective at achieving browser compatibility, how can you ensure a project maintains multi browser compatibility, and what framework is most
capable of delivering HTML5 enterprise applications?
When to deal with browser compatibility
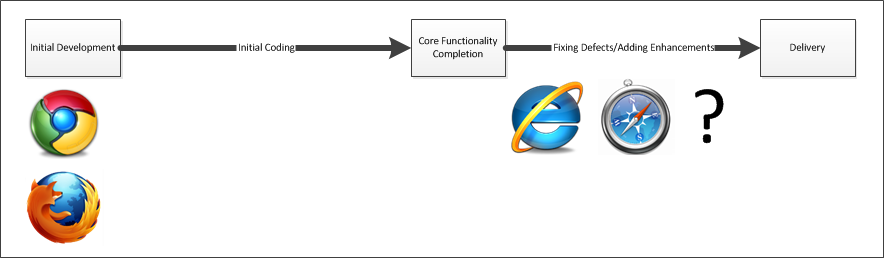
It is not recommended to deal with browser compatibility during the initial development of a new system. This is for the following reasons:
1. It adds a significant development overhead - When a developer works on some unit of initial functionality, they work on it in their development browser. If they have to support multiple browsers during the initial development, they are going to have to work on that same functionality that they normally would have worked out in one browser in several browsers. This obviously requires more time per unit of work.
2. Firefox and Chrome have better and faster debug capabilities - Include firebug in an HTML page that uses a debug version of a framework (such as ext-js-debug) and open that page in Firefox and then IE. You will notice that the page loads 10 times faster in Firefox. Chrome also has debug abilities natively, and in terms of Ext JS loads pages faster when using the debug framework.
3. Development time gets spent on maintenance - IE browser variants are incredibly easy to break doing things that don't cause problems in other browsers. A single trailing comma can cause an entire site to stop working in IE, and results in development time spent in chasing down something that is normally a maintenance activity.
4. Browser compatibility is easily dealt with after the fact (mostly) - Most compatibility issues are fairly simple, and therefore easily dealt with: trailing commas, console.log, bad css, and so on. It is when you start building highly customized non-standard components that browser compatibility needs to be considered beforehand.
5. Most compatibility issues come down to IE versus everything else - IE doesn't allow trailing commas, everything else does. IE doesn't support a lot of CSS 3 features, everything else does. IE doesn't provide a JavaScript console, most everything else does. What works in Chrome is highly likely to work in everything else but IE. Ultimately IE will be the browser with the compatibility issues, and is something that can be dealt with last.
The time for dealing with browser compatibility is at a milestone during the initial development where the system is mostly functionality, and the concentration moves from writing new code to fixing defects and adding enchancements. It is at this point that less code is generally being written, so it becomes less of a hassle to start figuring out why some things are not working in Internet Explorer.
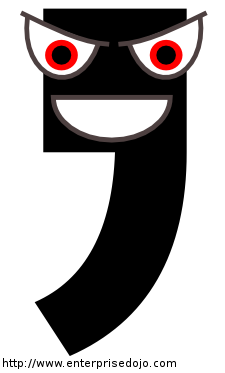
Trailing Commas
Trailing commas are a problem with Internet Explorer. These occur when a comma is left on the end of some list, which is acceptable in all browsers but Internet Explorer. The following are popular methods for defending against trailing commas:
- Development Environments: Tools like Komodo Edit catch trailing commas, but may not work with all frameworks.
- Plug-ins: The JDST Plug-in for Eclipse that comes with WTP 2+ will catch trailing commas, but only in certain frameworks and under certain conditions.
- Build Tools: Tools like JLint will detect trailing commas, but will not work out-of-the-box with frameworks like Ext JS.
- Discipline: Relying on developers to not add trailing commas themselves. A popular technique for doing this is to line break with the comma instead of after it when using lists.
Depending on the techniques used to defend against trailing commas, one will always make it through. At this point defense turns into offense. There are two types of trailing commas that produce different errors
1. Statically declared: These are trailing commas in lists that occur outside of the scope of the function, which are the most common. These are also the easiest to find, because in IE 8 or later you get a
"String or numbered undefined" error that includes a line number.
Example: {1,2,3,4,}
2. In a variable in a function: These are trailing commas in lists that occur inside of a variable declaration within a function, which are the hardest to find. In the case of a framework based language like Ext JS,
a line number of the error is provided, but it is at some location within the framework itself. In order to find the actual location you have to start with your primary view and start commenting out one custom component
at a time until the error goes away, and then start digging into the custom component.
Example: myFunction: function() { var a = {1,2,3,4,}; }
Console Logging
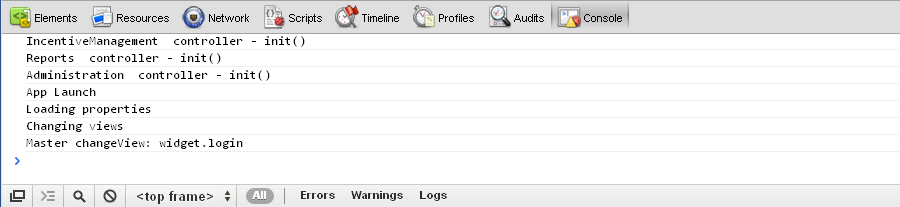
Console.log is a JavaScript function used to print-out information to the browser console. The problem with this is not all browsers have a console by default, so a console.log call in those browsers results in an error that breaks the application. In particular Firefox and Internet Explorer do not come equipped with a console, but this situation is easily dealt with in the following manner:
log: function(a) { try { console.log(a); } catch (er) { // do nothing } }
Define your own global log function that wraps the actual console.log in a try/catch block. In the event the browser doesn't support console.log, nothing happens.
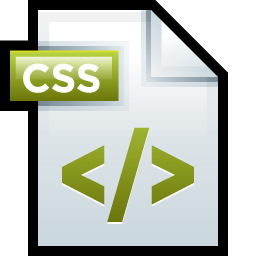
Styling
Styling a web application can be tricky business. The preferred means of styling web applications is using CSS, but again what works with CSS in one browser may not work in others (especially Internet Explorer).
This is where a framework like Ext JS/Sencha comes to the rescue. Since Internet Explorer and some mobile browsers do not handle common CSS activities like shadows, corner rounding, and transparency, Ext JS handles these browsers by using specialized stylesheets tailored to specific browsers.
ExtJS/Sencha works by using the idea of a theme. A language called SCSS is used to define the style of an application in a manner similar to CSS, and then the SCSS is used to generate the actual CSS to be used by the application. Once the CSS has been generated, images are "sliced" from the generated CSS to be used in browsers that don't support particular aspects of CSS.

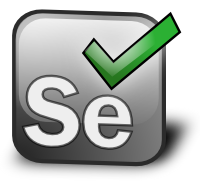
Testing
How does one "test" for browser compatibility? That all depends on the depth of testing to be undertaken. Testing can range from the following:
- Interaction testing of every component on every page on various browsers
- Seeing entry page of the application just displays on various browsers
Testing browser compatibility is a form of acceptance testing, as it usually requires a full-scale system test on the graphical user interface, which interacts with the server-side, which then interacts with the database. While the level of effort between testing every component on every page within an application and just testing the application's entry page is extremely large, the method for execution can be the same.
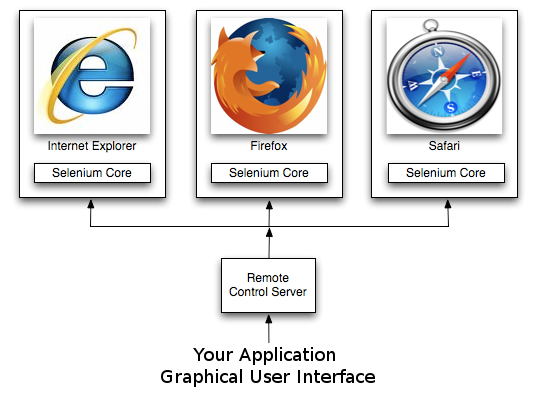
Selenium (http://seleniumhq.org) is a suite of tools for the automated driving of the graphical user interface of any application that displays in HTML format in a web browser. These tests can range from actions pre-recorded using the selenium web browser plug-in to tests written in Java code that execute as a JUnit test. The added advantage of this suite of tools is that it supports their execution in most every available type of web browser.
The Browser Compatibility Test
A sufficient browser compatibility test is one that just sees if the entry page of the application displays on various browsers. This is for the following reasons:
1. The most common compatibility problems result in the application not displaying at all - Trailing commas, console.log errors, and some CSS issues such as unsupported overlays all result in the same thing: the page doesn't display. The is an easy to implement method for preventing the all-to-common trailing comma from breaking an entire site for Internet Explorer.
2. Full test suites are better done after a significant portion of the system is complete - Full acceptance suites are vital for ensuring application stability, but that only applies for when the application itself has reached some point of stability. During an application's initial development it is under the most change, and trying to implement these level of tests during development adds a significant amount of overhead. This is because these tests have to be maintained, which is hard to do when the application is incomplete and being significantly changed all the time.
3. There is significantly less overhead with the entry page test - An entry page type test obviously has significantly less overhead. Worse case scenario is that you have to lookup and interact with a different field on the entry page.
4. The entry page test can apply to any system - An entry page test can simply look for a specified field on the page, and then repeat for any number of browsers. This logic applies to any system's entry page (which is typically a login page), making this generic test apply to any HTML based system.

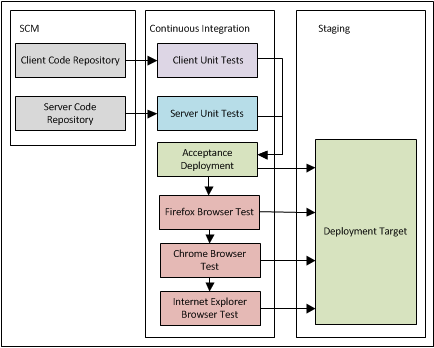
Browser Compatibility Testing
on Continuous Integration
The power of the browser compatibility test is with running it as a part of a system's continuous integration.
- Provides instant notification of a problem
- The problem is tied to a specific change set, making it easier to identify
- Prevents a broken site from being deployed to production
- Easily integrates into an existing acceptance test process
Ideally in an existing environment the browser compatibility tests would be applied either before or after the existing acceptance test suite. The only real requirement is that the browser compatibility test can only be run against a deployed system. For more information on what it takes to setup continuous integration, see http://www.appfoundation.com/ci/
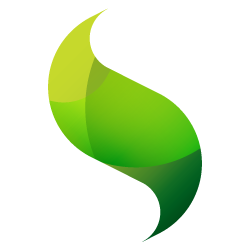
Technology Choice
Ext JS/Sencha has quite a few benefits in terms of browser compatibility:
- The theming handles all different browser types through CSS
- It is an enterprise MVC framework, that behaves the same across all browsers
- A trailing comma (in Internet Explorer) breaks the entire system, as opposed to just one page, making it easier to find
- Console.log (in non-supporting browsers) breaks the entire system, as opposed to just one page, making it easier to find
- It is HTML/JavaScript based, so tools like Selenium easily work with it
- It has specific packaging for mobile applications for the Apple AppStore and Android Market Place
In combination with the browser compatibility test, Ext JS/Sencha is a good framework for creating HTML5 based applications and keeping them working in all browsers. It should be noted through that the browser compatibility test described works with any HTML/JavaScript based technology. The browser compatibility test is also more of a smoke test, and is not intended to replace more detailed acceptance tests. It is intended as an easy to implement and maintain method for catching the majority of common compatibility issues, in which it has proven as very effective
Ready to Start Testing Browser Compatibility?
AppFoundation has also created its own browser compatibility test that is ready to run on any Continuous Integration system, which is further described at framework.appfoundation.com. In addition, for further customized solutions contact AppFoundation.